Qt Signal Slot Template Class

- Qt Signal Slot Template Classic
- Qt Signal Slot Template Classification
- Qt Signal Slot Template Classifieds
- Qt Signal Slot Template Classes
Qt Signal Slot Template Classic
template <typename T> class QFutureWatcherThe QFutureWatcher class allows monitoring a QFuture using signals and slots. More...
Each PyQt widget, which is derived from QObject class, is designed to emit ‘signal’ in response to one or more events. The signal on its own does not perform any action. Instead, it is ‘connected’ to a ‘slot’. The slot can be any callable Python function. In PyQt, connection between a signal and a slot can be achieved in different ways. QtCore.SIGNAL and QtCore.SLOT macros allow Python to interface with Qt signal and slot delivery mechanisms. This is the old way of using signals and slots. The example below uses the well known clicked signal from a QPushButton. The connect method has a non python-friendly syntax. So I'd like to make a template class in C Qt. The problem is that I'm using signals/slots in this class. My class header looks like template class T class Container: public QObject.
Header: | #include <QFutureWatcher> |
CMake: | find_package(Qt6 COMPONENTS Core REQUIRED) target_link_libraries(mytarget PRIVATE Qt6::Core) |
qmake: | QT += core |
Inherits: | QObject |
Note: All functions in this class are reentrant.
Public Functions
QFutureWatcher(QObject *parent = nullptr) | |
virtual | ~QFutureWatcher() |
QFuture<T> | future() const |
bool | isCanceled() const |
bool | isFinished() const |
bool | isRunning() const |
bool | isStarted() const |
bool | isSuspended() const |
bool | isSuspending() const |
int | progressMaximum() const |
int | progressMinimum() const |
QString | progressText() const |
int | progressValue() const |
T | result() const |
T | resultAt(int index) const |
void | setFuture(const QFuture<T> &future) |
void | setPendingResultsLimit(int limit) |
void | waitForFinished() |
Public Slots
void | cancel() |
void | resume() |
void | setSuspended(bool suspend) |
void | suspend() |
void | toggleSuspended() |
Signals
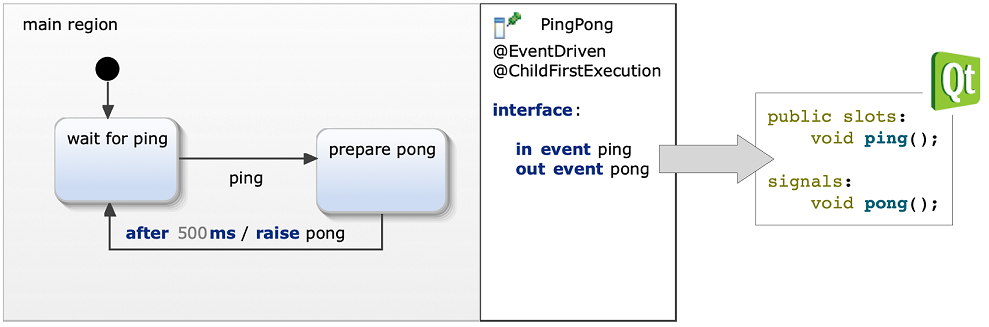
void | canceled() |
void | finished() |
void | progressRangeChanged(int minimum, int maximum) |
void | progressTextChanged(const QString &progressText) |
void | progressValueChanged(int progressValue) |
void | resultReadyAt(int index) |
void | resultsReadyAt(int beginIndex, int endIndex) |
void | resumed() |
void | started() |
void | suspended() |
void | suspending() |
Detailed Description
QFutureWatcher provides information and notifications about a QFuture. Use the setFuture() function to start watching a particular QFuture. The future() function returns the future set with setFuture().
For convenience, several of QFuture's functions are also available in QFutureWatcher: progressValue(), progressMinimum(), progressMaximum(), progressText(), isStarted(), isFinished(), isRunning(), isCanceled(), isSuspending(), isSuspended(), waitForFinished(), result(), and resultAt(). The cancel(), setSuspended(), suspend(), resume(), and toggleSuspended() functions are slots in QFutureWatcher.
Status changes are reported via the started(), finished(), canceled(), suspending(), suspended(), resumed(), resultReadyAt(), and resultsReadyAt() signals. Progress information is provided from the progressRangeChanged(), void progressValueChanged(), and progressTextChanged() signals.
Throttling control is provided by the setPendingResultsLimit() function. When the number of pending resultReadyAt() or resultsReadyAt() signals exceeds the limit, the computation represented by the future will be throttled automatically. The computation will resume once the number of pending signals drops below the limit.
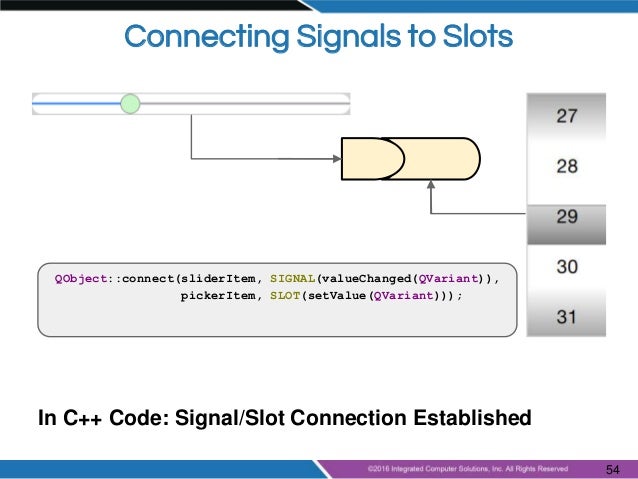
Example: Starting a computation and getting a slot callback when it's finished:
Be aware that not all running asynchronous computations can be canceled or suspended. For example, the future returned by QtConcurrent::run() cannot be canceled; but the future returned by QtConcurrent::mappedReduced() can.
QFutureWatcher<void> is specialized to not contain any of the result fetching functions. Any QFuture<T> can be watched by a QFutureWatcher<void> as well. This is useful if only status or progress information is needed; not the actual result data.
See also QFuture and Qt Concurrent.
Member Function Documentation
QFutureWatcher::QFutureWatcher(QObject *parent = nullptr)
Constructs a new QFutureWatcher with the given parent. Until a future is set with setFuture(), the functions isStarted(), isCanceled(), and isFinished() return true
.
[slot]
void QFutureWatcher::cancel()
Cancels the asynchronous computation represented by the future(). Note that the cancelation is asynchronous. Use waitForFinished() after calling cancel() when you need synchronous cancelation.
Currently available results may still be accessed on a canceled QFuture, but new results will not become available after calling this function. Also, this QFutureWatcher will not deliver progress and result ready signals once canceled. This includes the progressValueChanged(), progressRangeChanged(), progressTextChanged(), resultReadyAt(), and resultsReadyAt() signals.
Be aware that not all running asynchronous computations can be canceled. For example, the QFuture returned by QtConcurrent::run() cannot be canceled; but the QFuture returned by QtConcurrent::mappedReduced() can.
[signal]
void QFutureWatcher::canceled()
This signal is emitted if the watched future is canceled.
[signal]
void QFutureWatcher::finished()
This signal is emitted when the watched future finishes.
[signal]
void QFutureWatcher::progressRangeChanged(intminimum, intmaximum)
The progress range for the watched future has changed to minimum and maximum
[signal]
void QFutureWatcher::progressTextChanged(const QString &progressText)
This signal is emitted when the watched future reports textual progress information, progressText.
[signal]
void QFutureWatcher::progressValueChanged(intprogressValue)
This signal is emitted when the watched future reports progress, progressValue gives the current progress. In order to avoid overloading the GUI event loop, QFutureWatcher limits the progress signal emission rate. This means that listeners connected to this slot might not get all progress reports the future makes. The last progress update (where progressValue equals the maximum value) will always be delivered.
[signal]
void QFutureWatcher::resultReadyAt(intindex)
This signal is emitted when the watched future reports a ready result at index. If the future reports multiple results, the index will indicate which one it is. Results can be reported out-of-order. To get the result, call resultAt(index);
[signal]
void QFutureWatcher::resultsReadyAt(intbeginIndex, intendIndex)
This signal is emitted when the watched future reports ready results. The results are indexed from beginIndex to endIndex.
[slot]
void QFutureWatcher::resume()
Resumes the asynchronous computation represented by the future(). This is a convenience method that simply calls setSuspended(false).
See also suspend().
[signal]
void QFutureWatcher::resumed()
This signal is emitted when the watched future is resumed.
[slot, since 6.0]
void QFutureWatcher::setSuspended(boolsuspend)
If suspend is true, this function suspends the asynchronous computation represented by the future(). If the computation is already suspended, this function does nothing. QFutureWatcher will not immediately stop delivering progress and result ready signals when the future is suspended. At the moment of suspending there may still be computations that are in progress and cannot be stopped. Signals for such computations will still be delivered.
If suspend is false, this function resumes the asynchronous computation. If the computation was not previously suspended, this function does nothing.
Be aware that not all computations can be suspended. For example, the QFuture returned by QtConcurrent::run() cannot be suspended; but the QFuture returned by QtConcurrent::mappedReduced() can.
This function was introduced in Qt 6.0.
See also suspended(), suspend(), resume(), and toggleSuspended().
[signal]
void QFutureWatcher::started()
This signal is emitted when this QFutureWatcher starts watching the future set with setFuture().
[slot, since 6.0]
void QFutureWatcher::suspend()
Suspends the asynchronous computation represented by this future. This is a convenience method that simply calls setSuspended(true).
Qt Signal Slot Template Classification
This function was introduced in Qt 6.0.
See also resume().
[signal, since 6.0]
void QFutureWatcher::suspended()
This signal is emitted when suspend() took effect, meaning that there are no more running computations. After receiving this signal no more result ready or progress reporting signals are expected.
This function was introduced in Qt 6.0.
See also setSuspended(), suspend(), and suspended().
[signal, since 6.0]
void QFutureWatcher::suspending()
This signal is emitted when the state of the watched future is set to suspended.
Note: This signal only informs that suspension has been requested. It doesn't indicate that all background operations are stopped. Signals for computations that were in progress at the moment of suspending will still be delivered. To be informed when suspension actually took effect, use the suspended() signal.
This function was introduced in Qt 6.0.
See also setSuspended(), suspend(), and suspended().
[slot, since 6.0]
void QFutureWatcher::toggleSuspended()
Toggles the suspended state of the asynchronous computation. In other words, if the computation is currently suspending or suspended, calling this function resumes it; if the computation is running, it is suspended. This is a convenience method for calling setSuspended(!(isSuspending() isSuspended())).
This function was introduced in Qt 6.0.
See also setSuspended(), suspend(), and resume().
[virtual]
QFutureWatcher::~QFutureWatcher()
Destroys the QFutureWatcher.
QFuture<T> QFutureWatcher::future() const
Returns the watched future.
See also setFuture().
bool QFutureWatcher::isCanceled() const
Returns true
if the asynchronous computation has been canceled with the cancel() function, or if no future has been set; otherwise returns false
.
Be aware that the computation may still be running even though this function returns true
. See cancel() for more details.
bool QFutureWatcher::isFinished() const
Returns true
if the asynchronous computation represented by the future() has finished, or if no future has been set; otherwise returns false
.
bool QFutureWatcher::isRunning() const
Returns true
if the asynchronous computation represented by the future() is currently running; otherwise returns false
.
bool QFutureWatcher::isStarted() const
Returns true
if the asynchronous computation represented by the future() has been started, or if no future has been set; otherwise returns false
.
[since 6.0]
bool QFutureWatcher::isSuspended() const
Returns true
if a suspension of the asynchronous computation has been requested, and it is in effect, meaning that no more results or progress changes are expected.
This function was introduced in Qt 6.0.
See also suspended(), setSuspended(), and isSuspending().
[since 6.0]
bool QFutureWatcher::isSuspending() const
Returns true
if the asynchronous computation has been suspended with the suspend() function, but the work is not yet suspended, and computation is still running. Returns false
otherwise.
To check if suspension is actually in effect, use isSuspended() instead.
This function was introduced in Qt 6.0.
See also setSuspended(), toggleSuspended(), and isSuspended().
int QFutureWatcher::progressMaximum() const
Returns the maximum progressValue().
See also progressValue() and progressMinimum().
int QFutureWatcher::progressMinimum() const
Returns the minimum progressValue().
See also progressValue() and progressMaximum().
QString QFutureWatcher::progressText() const
Returns the (optional) textual representation of the progress as reported by the asynchronous computation.
Be aware that not all computations provide a textual representation of the progress, and as such, this function may return an empty string.
int QFutureWatcher::progressValue() const
Returns the current progress value, which is between the progressMinimum() and progressMaximum().
See also progressMinimum() and progressMaximum().
template <typename U, typename> T QFutureWatcher::result() const
Returns the first result in the future(). If the result is not immediately available, this function will block and wait for the result to become available. This is a convenience method for calling resultAt(0).
See also resultAt().
template <typename U, typename> T QFutureWatcher::resultAt(intindex) const
Returns the result at index in the future(). If the result is not immediately available, this function will block and wait for the result to become available.
See also result().
void QFutureWatcher::setFuture(const QFuture<T> &future)
Starts watching the given future.
One of the signals might be emitted for the current state of the future. For example, if the future is already stopped, the finished signal will be emitted.
To avoid a race condition, it is important to call this function after doing the connections.
See also future().
void QFutureWatcher::setPendingResultsLimit(intlimit)
The setPendingResultsLimit() provides throttling control. When the number of pending resultReadyAt() or resultsReadyAt() signals exceeds the limit, the computation represented by the future will be throttled automatically. The computation will resume once the number of pending signals drops below the limit.
void QFutureWatcher::waitForFinished()
Waits for the asynchronous computation to finish (including cancel()ed computations), i.e. until isFinished() returns true
.
© 2020 The Qt Company Ltd. Documentation contributions included herein are the copyrights of their respective owners. The documentation provided herein is licensed under the terms of the GNU Free Documentation License version 1.3 as published by the Free Software Foundation. Qt and respective logos are trademarks of The Qt Company Ltd. in Finland and/or other countries worldwide. All other trademarks are property of their respective owners.
How often is a an object copied, if it is emitted by a signal as a const reference and received by a slot as a const reference? How does the behaviour differ for direct and queued signal-slot connections? What changes if we emit the object by value or receive it by value?
Nearly every customer asks this question at some point in a project. The Qt documentation doesn’t say a word about it. There is a good discussion on stackoverflow, which unfortunately leaves it to the reader to pick the right answer from all the answers and comments. So, let’s have a systematic and detailed look at how arguments are passed to signals and slots.
Setting the Stage
For our experiments, we need a copyable class that we will pass by const reference or by value to signals and slots. The class – let’s call it Copy
– looks as follows.
The copy constructor and the assignment operator simply perform a member-wise copy – like the compiler generated versions would do. We implement them explicitly to set breakpoints or to print debugging messages. The default constructor is only required for queued connections. We’ll learn the reason later.
We need another class, MainView
, which ultimately derives from QObject
. MainView provides the following signals and slots.
MainView
provides four signal-slot connections for each connection type.
The above code is used for direct connections. For queued connections, we comment out the first line and uncomment the second and third line.
The code for emitting the signals looks as follows:
Direct Connections
sendConstRef => receiveConstRef
We best set breakpoints in the copy constructor and assignment operator of the Copy
class. If our program only calls emit sendConstRef(c)
, the breakpoints are not hit at all. So, no copies happen. Why?
The result is not really surprising, because this is exactly how passing arguments as const references in C++ works and because a direct signal-slot connection is nothing else but a chain of synchronous or direct C++ function calls.
Nevertheless, it is instructive to look at the chain of function calls executed when the sendConstRef
signal is emitted.
The meta-object code of steps 2, 3 and 4 – for marshalling the arguments of a signal, routing the emitted signal to the connected slots and de-marshalling the arguments for the slot, respectively – is written in such a way that no copying of the arguments occurs. This leaves us with two places, where copying of a Copy
object could potentially occur: when passing the Copy
object to the functions MainView::sendConstRef
or MainView::receiveConstRef
.
These two places are governed by standard C++ behaviour. Copying is not needed, because both functions take their arguments as const references. There are also no life-time issues for the Copy
object, because receiveConstRef
returns before the Copy
object goes out of scope at the end of sendConstRef
.
sendConstRef => receiveValue
Based on the detailed analysis in the last section, we can easily figure out that only one copy is needed in this scenario. When qt_static_meta_call
calls receiveValue(Copy c)
in step 4, the original Copy
object is passed by value and hence must be copied.
Qt Signal Slot Template Classifieds
sendValue => receiveConstRef
One copy happens, when the Copy
object is passed by value to sendValue
by value.
sendValue => receiveValue
This is the worst case. Two copies happen, one when the Copy
object is passed to sendValue
by value and another one when the Copy
object is passed to receiveValue
by value.
Queued Connections
A queued signal-slot connection is nothing else but an asynchronous function call. Conceptually, the routing function QMetaObject::activate
does not call the slot directly any more, but creates a command object from the slot and its arguments and inserts this command object into the event queue. When it is the command object’s turn, the dispatcher of the event loop will remove the command object from the queue and execute it by calling the slot.
When QMetaObject::activate
creates the command object, it stores a copy of the Copy
object in the command object. Therefore, we have one extra copy for every signal-slot combination.
We must register the Copy
class with Qt’s meta-object system with the command qRegisterMetaType('Copy');
in order to make the routing of QMetaObject::activate
work. Any meta type is required to have a public default constructor, copy constructor and destructor. That’s why Copy
has a default constructor.
Queued connections do not only work for situations where the sender of the signal and the receiver of the signal are in the same thread, but also when the sender and receiver are in different threads. Even in a multi-threaded scenario, we should pass arguments to signals and slots by const reference to avoid unnecessary copying of the arguments. Qt makes sure that the arguments are copied before they cross any thread boundaries.
Conclusion
The following table summarises our results. The first line, for example, reads as follows: If the program passes the argument by const reference to the signal and also by const reference to the slot, there are no copies for a direct connection and one copy for a queued connection.
Qt Signal Slot Template Classes
Signal | Slot | Direct | Queued |
---|---|---|---|
const Copy& | const Copy& | 0 | 1 |
const Copy& | Copy | 1 | 2 |
Copy | const Copy& | 1 | 2 |
Copy | Copy | 2 | 3 |
The conclusion from the above results is that we should pass arguments to signals and slots by const reference and not by value. This advice is true for both direct and queued connections. Even if the sender of the signal and the receiver of the slot are in different threads, we should still pass arguments by const reference. Qt takes care of copying the arguments, before they cross the thread boundaries – and everything is fine.
By the way, it doesn’t matter whether we specify the argument in a connect call as const Copy&
or Copy
. Qt normalises the type to Copy
any way. This normalisation does not imply, however, that arguments of signals and slots are always copied – no matter whether they are passed by const reference or by value.